When creating Wear OS by Google apps for China, you need to account for handsets without Google Play services pre-installed. This page contains common changes that international developers may need to adopt for the Chinese market.
Use the correct version of Google Play services
Google Play services version 10.2.0 provides worldwide support for the Fused Location Provider API and the Data Layer API. Developers must use this version if they use these APIs. In other cases this dependency is optional.
Fused Location Provider API
If you use the Fused Location Provider API, you need to include the following dependency in
the build.gradle
file of your wear module:
dependencies {
...
compile 'com.google.android.gms:play-services-location:10.2.0'
}
Data Layer API
Important: Using the Data Layer API is no longer recommended since Wear 2.0. This is because apps can now access the internet directly, which simplifies code development. In addition, iOS-paired Wear devices don't support the Data Layer API.
If your app uses the Data Layer API, you need to add the following line to the
build.gradle
file of your wear module. The line requires use of the 10.2.0 version of
the client library:
dependencies {
...
compile 'com.google.android.gms:play-services-wearable:10.2.0'
...
}
In addition, you need to add the following line to the build.gradle
file of
your mobile module. Replace the Google Play services dependency with a reference to the
10.2.0 version.
dependencies {
...
compile 'com.google.android.gms:play-services-wearable:10.2.0'
}
Authentication
Before implementing authentication, review your use cases to see if authentication is actually needed. For example, for an app delivering the weather forecast, there likely is no need for sign-in and thus for authentication.
If you do require authentication, we recommend using OAuth 2.0 or, as a fall back measure, on-device input. Alternatively, you can pass a security token using the Data Layer. However, using the Data Layer is not recommended as it doesn't support Wear OS by Google devices paired with iOS devices.
Bridged notifications
Bridged notifications are not supported in China. Phone notifications are bridged to Wear OS by Google only if the Wear device is connected to the phone via bluetooth.
Location and mapping coordinates compatibility
You should use the FusedLocationProvider to detect the user's location in China, in the same way as for the rest of the world. This ensures that your app takes into account the best information regardless of the watch hardware and the phone platform that the watch is paired to. In addition, there will be benefits from battery optimization that is built into the Wear OS by Google platform.
When integrating FusedLocationProvider
with third-party map SDKs, you
should take into account the coordinates compatibility among providers.
FusedLocationProvider
reports the location according to the
WGS84 standard. Be sure to
convert coordinate systems, as appropriate.
Google Fit support
Google Fit's accumulated step counter is supported in China, with up to seven days of history. You can access this without providing a user credential.
Emulator support
You can use the China version of the Wear OS by Google emulator image to test your apps. This is supported by Android Studio 3.0 and above.
To test your apps on the China version of the emulator:
- Ensure that you have installed the Android Emulator 26.1.2 version.
- Download the Wear OS by Google for China images from the SDK manager.
- Choose to use the Wear OS by Google for China image when creating an AVD profile.
- Run the Wear OS by Google for China emulator for development.
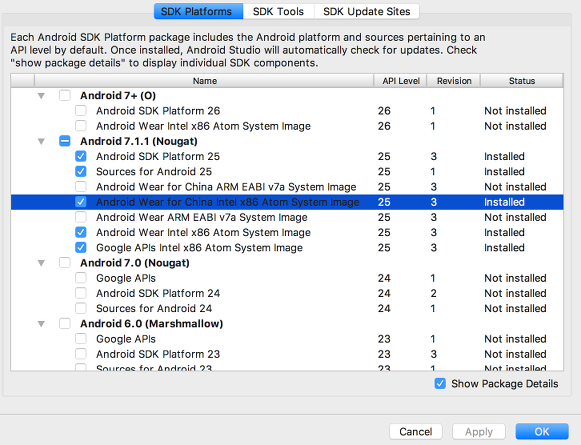
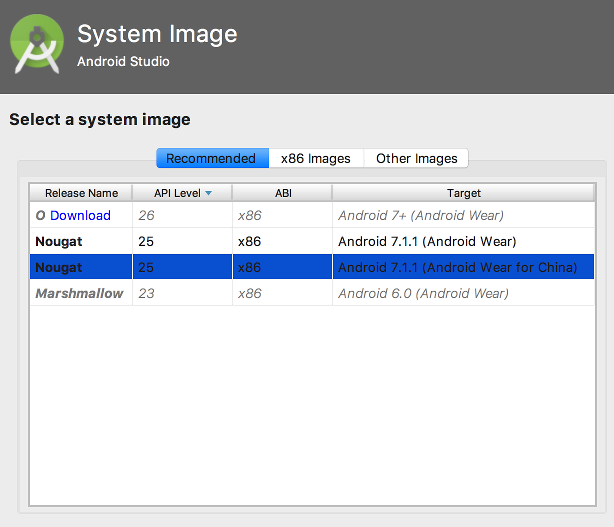
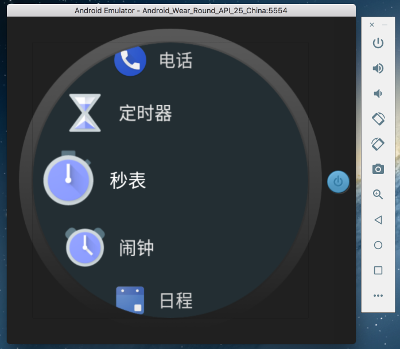
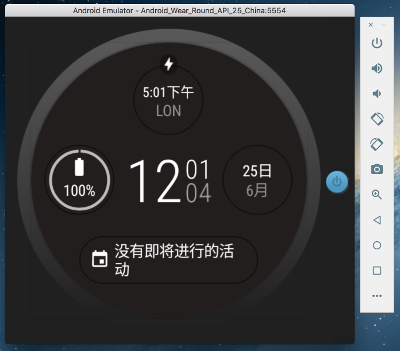
Initiate an app-specific Bluetooth and Wi-Fi channel
Wear OS by Google automatically routes network requests. In most cases, there's no requirement for the app to open an app-specific Bluetooth and Wi-Fi channel.
If an app does request an app-specific Bluetooth and Wi-Fi channel in China, the request will
silently fail. A pop-up dialog is displayed
asking the user for confirmation. If the user confirms, the channel is opened. This happens every
time (i.e. not just on first use) BluetoothAdapter.enable()
or
WifiManager.setEnabled(true)
is
called.
Permission Review Mode
In China, Wear OS by Google for China devices run in Permission Review Mode
, which
imposes some limits on how apps with a targetApiLevel
lower than 23 can be used:
- Even though permissions are granted at installation time, when an app with a
targetApiLevel
lower than 23 starts for the first time, a dialog appears asking the user to confirm permissions for this app. - All components in the app, such as broadcast receivers, services, activities, etc., would not respond to corresponding events before the app is used for the first time.
As a result, we strongly recommend that you use targetApiLevel
23 or above
and adopt the
runtime permissions
best practices.
Use other Google Play services APIs
If your app uses Google Play services APIs other than the Wearable API, then your app needs to check whether these APIs are available to use during runtime and respond appropriately. There are two ways to check the availability of Google Play service APIs:
- Use a separate GoogleApiClient instance for connecting to other APIs. This interface contains callbacks to alert your app to the success or failure of the connection. In the case of failed connection, the ConnectionResult will show API_UNAVAILABLE. To learn how to handle connection failures, see Accessing Google APIs.
- Use the addApiIfAvailable() method of GoogleApiClient.Builder to connect to the required APIs. After the onConnected() callback fires, use the hasConnectedApi() method to ensure that each of the requested APIs is connected correctly.